How To Format a Date as DD/MM/YYYY in JavaScript
Blog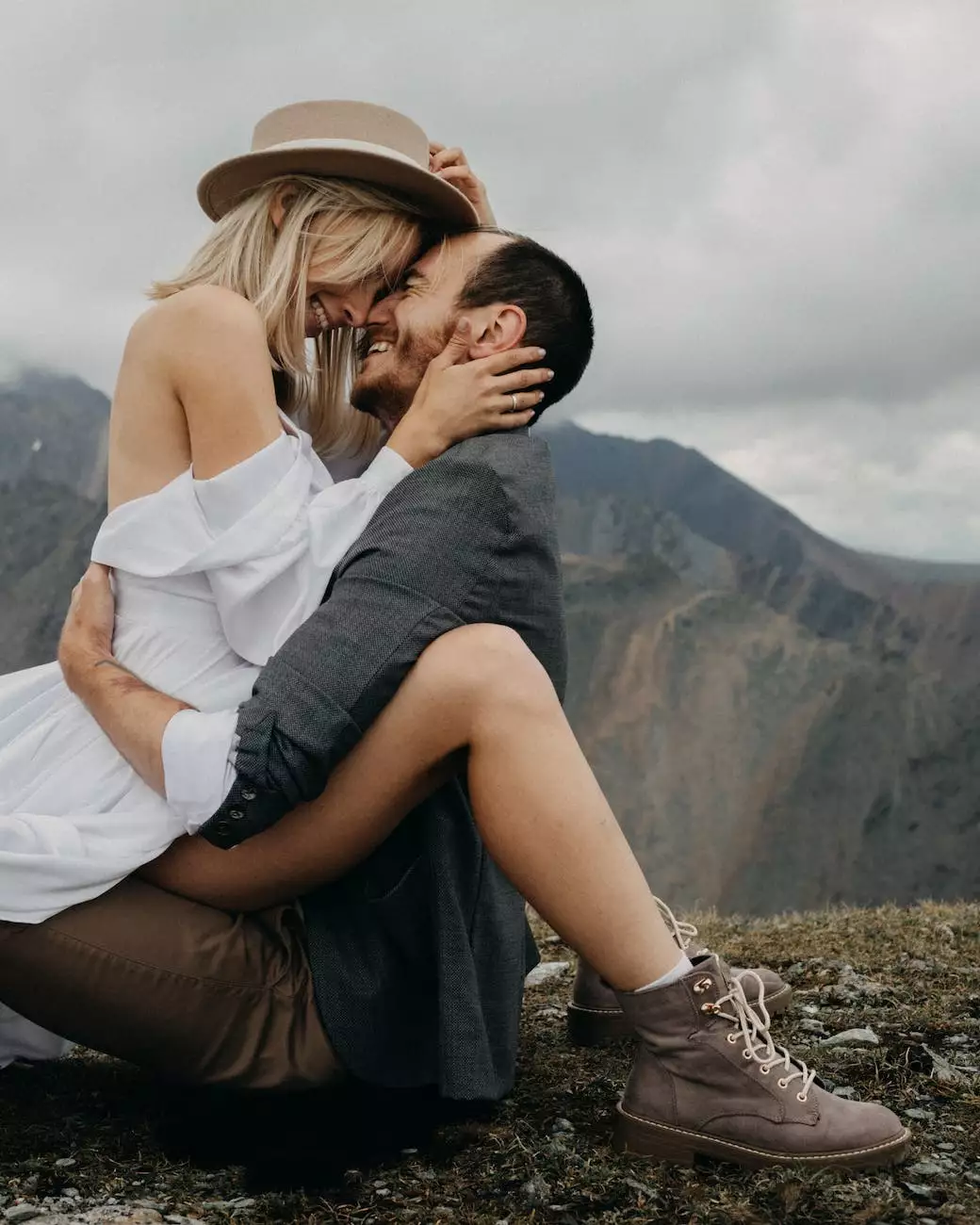
Introduction
Welcome to Genevish Graphics, your go-to resource for all things arts and entertainment in the visual arts and design industry. In this guide, we will explore the intricacies of formatting dates as DD/MM/YYYY using JavaScript, helping you enhance your web development projects with elegant date displays.
Understanding Date Formatting
Before we dive into the specifics of formatting dates in JavaScript, let's briefly understand the importance of proper date formatting. Dates play a vital role in various web applications, ranging from event management systems to online booking platforms. By formatting dates correctly, you ensure that users can interpret and interact with them accurately, enhancing the overall user experience.
Why Choose JavaScript?
JavaScript is a popular programming language widely used for web development. It offers robust date handling capabilities, allowing developers to manipulate and format dates effortlessly. By leveraging JavaScript's built-in date functions and methods, you can easily customize date formats according to your requirements.
The DD/MM/YYYY Format
The DD/MM/YYYY format, commonly used in many parts of the world, presents dates in a day-month-year sequence. This format is intuitive and ensures clarity for users. Now, let's take a closer look at how you can achieve this formatting in JavaScript.
Formatting Dates in JavaScript
To format a date as DD/MM/YYYY in JavaScript, you can utilize the following steps:
- Create a new Date object using the desired date.
- Extract the day, month, and year components from the Date object.
- Concatenate the day, month, and year components with appropriate separators.
- Display the formatted date to the user.
Let's see an example:
// Create a new Date object const currentDate = new Date(); // Extract day, month, and year components const day = String(currentDate.getDate()).padStart(2, '0'); const month = String(currentDate.getMonth() + 1).padStart(2, '0'); const year = currentDate.getFullYear(); // Format the date as DD/MM/YYYY const formattedDate = `${day}/${month}/${year}`; // Display the formatted date console.log(formattedDate);By following these simple steps, you can effortlessly format any date as DD/MM/YYYY in JavaScript. Feel free to incorporate this code into your web applications for seamless date formatting.
Additional Tips and Best Practices
While date formatting may appear straightforward, there are a few additional tips and best practices to consider:
- Locale Considerations: Keep in mind that date formats can vary across different countries and regions. Ensure that your code adapts to the user's locale.
- Consistency: Maintain consistency in date formats throughout your application to avoid confusion among users.
- Error Handling: Implement proper error handling to gracefully handle situations where invalid or unexpected dates are encountered.
By adhering to these tips, you ensure that your date formatting implementation remains robust and user-friendly.
Conclusion
Congratulations! You now have a comprehensive understanding of how to format a date as DD/MM/YYYY in JavaScript. Armed with this knowledge, you can enhance the visual appeal and functionality of your web development projects. Remember to refer back to this guide whenever you need a quick refresher or assistance with date formatting.
Genevish Graphics is dedicated to providing valuable resources and solutions in the arts and entertainment industry. With our expertise in visual arts and design, we are committed to empowering creative individuals like you.
Explore more informative guides and articles on our website to take your arts and entertainment endeavors to new heights. Happy coding!