Cannot read property 'replace' of Undefined in JS - Isotropic
Blog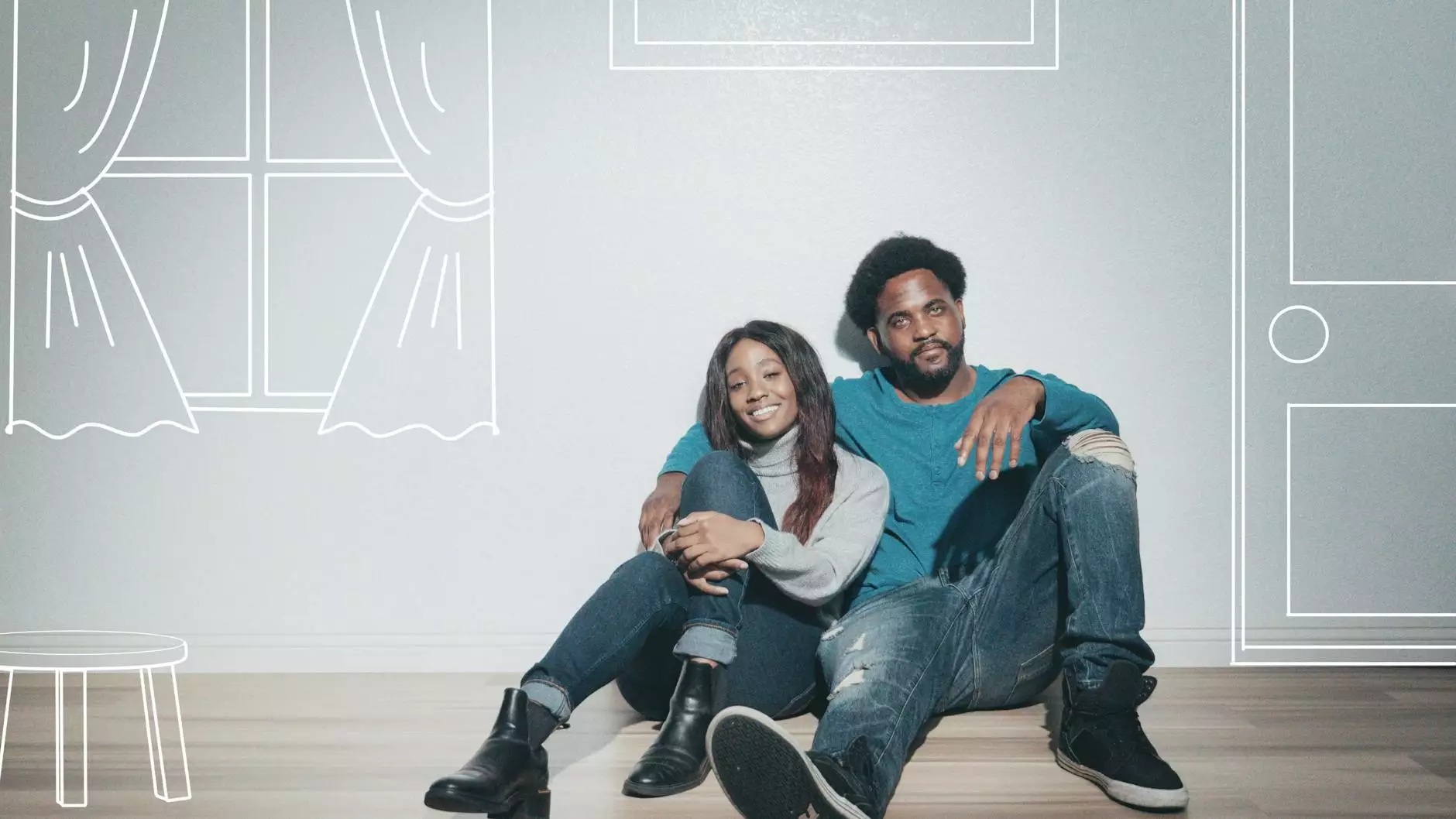
Welcome to Genevish Graphics, your go-to resource for Arts & Entertainment in the field of Visual Arts and Design. In this article, we will dive into a common error encountered in JavaScript development - "Cannot read property 'replace' of Undefined." Whether you are a beginner or an experienced developer, understanding and resolving this error is crucial for building robust and error-free web applications.
What is the "Cannot read property 'replace' of Undefined" error?
When working with JavaScript, you may come across an error message similar to "Cannot read property 'replace' of Undefined." This error typically occurs when you attempt to use the replace() method on a variable or object that is undefined or null. The replace() method is used to replace occurrences of a specified value with another value in a string, but it can only be called on a valid string object.
Causes of the "Cannot read property 'replace' of Undefined" error
There are a few common scenarios that can lead to this error:
- Undefined or null variable: If you try to call the replace() method on a variable that is either undefined or null, the error will occur. To resolve this, make sure the variable is properly initialized and not null before attempting to use the replace() method.
- Undefined or null object property: If you attempt to access an object property that is undefined or null, and then try to use the replace() method on it, the error will occur. Ensure that the object property exists and has a valid value before using the replace() method.
- Incorrect usage of the replace() method: It is also possible to encounter this error if you misuse the replace() method itself. Double-check your syntax and make sure you are using the method correctly, providing the necessary arguments and ensuring that the target string is valid.
How to fix the "Cannot read property 'replace' of Undefined" error
Now that we understand the causes of this error, let's explore some solutions:
1. Check variable or object initialization
If you are encountering this error due to an undefined or null variable or object property, you can prevent it by ensuring proper initialization:
let myString = ""; // Initialize the variable with an empty string myString = myString.replace("foo", "bar"); // Perform the replace operationBy initializing the variable with a valid value, such as an empty string, you can avoid the error and safely use the replace() method.
2. Validate object properties
When working with objects, it's important to validate the properties before using the replace() method:
let myObject = { text: null, // Assuming the text property is null }; if (myObject.text !== null) { myObject.text = myObject.text.replace("foo", "bar"); // Perform the replace operation }By checking if the object property is not null, you can avoid the error and safely use the replace() method on a valid string.
3. Review replace() method usage
If you have verified that the variable or object is properly initialized, and you are still encountering the error, review your usage of the replace() method itself:
let myString = "Hello, world!"; myString = myString.replace("foo", "bar"); // Incorrect usage - "foo" not found in myStringMake sure that the target string contains the value you are trying to replace. If the specified value doesn't exist in the string, the replace() method will not make any changes and won't throw an error.
Conclusion
In conclusion, the "Cannot read property 'replace' of Undefined" error is a common issue in JavaScript development. By properly initializing variables and validating object properties, as well as ensuring correct usage of the replace() method, you can avoid this error and create reliable and error-free JavaScript applications.
Thank you for choosing Genevish Graphics as your resource for Arts & Entertainment in the Visual Arts and Design category. Feel free to explore our website for more information and helpful resources.