What is the Most Efficient Way to groupby Objects in an Array?
Blog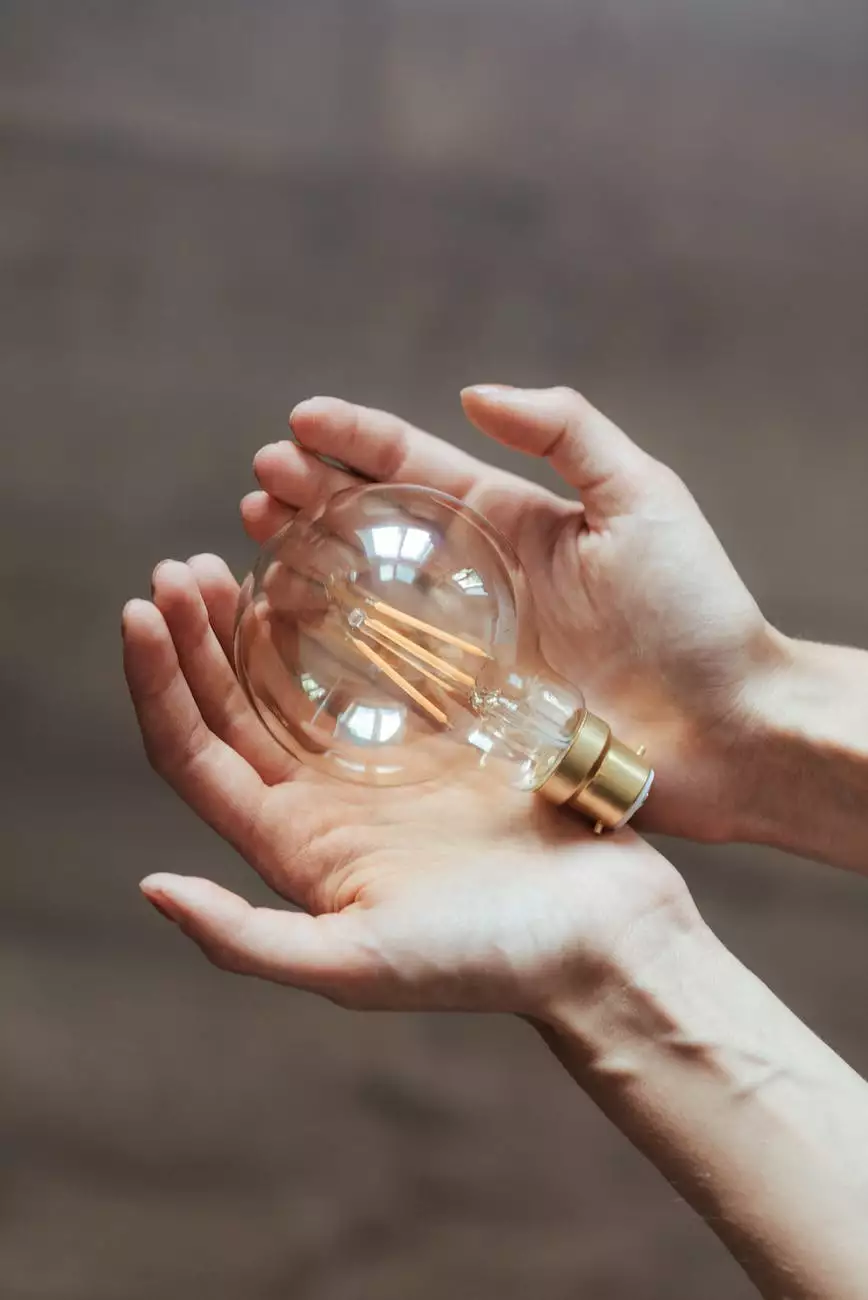
Introduction
Welcome to Genevish Graphics, your ultimate resource for arts and entertainment in the field of visual arts and design. In this tutorial, we will explore the most efficient way to group objects in an array using JavaScript.
Understanding the GroupBy Functionality
In JavaScript, arrays are a fundamental data structure that allows you to store multiple values. Often, you may come across a scenario where you need to group objects within an array based on a common property value. This is where the GroupBy functionality becomes invaluable.
Traditional Approach
Before diving into the most efficient way to group objects in an array, let's explore the traditional approach. Typically, developers would use loops and conditional statements to iterate over the array and create separate groups manually. While this approach works, it can be time-consuming and error-prone.
Introducing the New Approach
Fortunately, there is a more efficient and elegant way to achieve this using JavaScript's built-in methods. The new approach leverages the power of the reduce and map functions, allowing you to achieve the desired grouping swiftly and with minimal code.
Step-by-Step Guide
Step 1: Prepare Your Data
The first step is to ensure your data is structured correctly. Make sure your array consists of objects with a common property that you want to group by. For example, if you have an array of products, each product should have a "category" property.
Step 2: Implement the GroupBy Function
Now, let's dive into the implementation. First, create a function called groupBy that takes in two parameters: the array you want to group and the property to group by.
function groupBy(array, property) { return array.reduce((acc, obj) => { const key = obj[property]; if (!acc[key]) { acc[key] = []; } acc[key].push(obj); return acc; }, {}); }Step 3: Test Your GroupBy Function
After implementing the groupBy function, it's time to test it with your data. Call the function, passing in your array and the property you wish to group by.
const products = [ { name: "Product 1", category: "Category A" }, { name: "Product 2", category: "Category B" }, { name: "Product 3", category: "Category A" }, { name: "Product 4", category: "Category C" }, // Additional products here... ]; const groupedProducts = groupBy(products, "category"); console.log(groupedProducts);Conclusion
Congratulations! You have successfully learned the most efficient way to group objects in an array using JavaScript. This new approach will save you time and effort, allowing you to manipulate your data in a structured manner.
At Genevish Graphics, we strive to provide comprehensive tutorials and resources to help you master various visual arts and design techniques. Stay tuned for more informative content to enhance your creative skills!