How To Fix Cannot Read Property 'style' of Null in JavaScript
Blog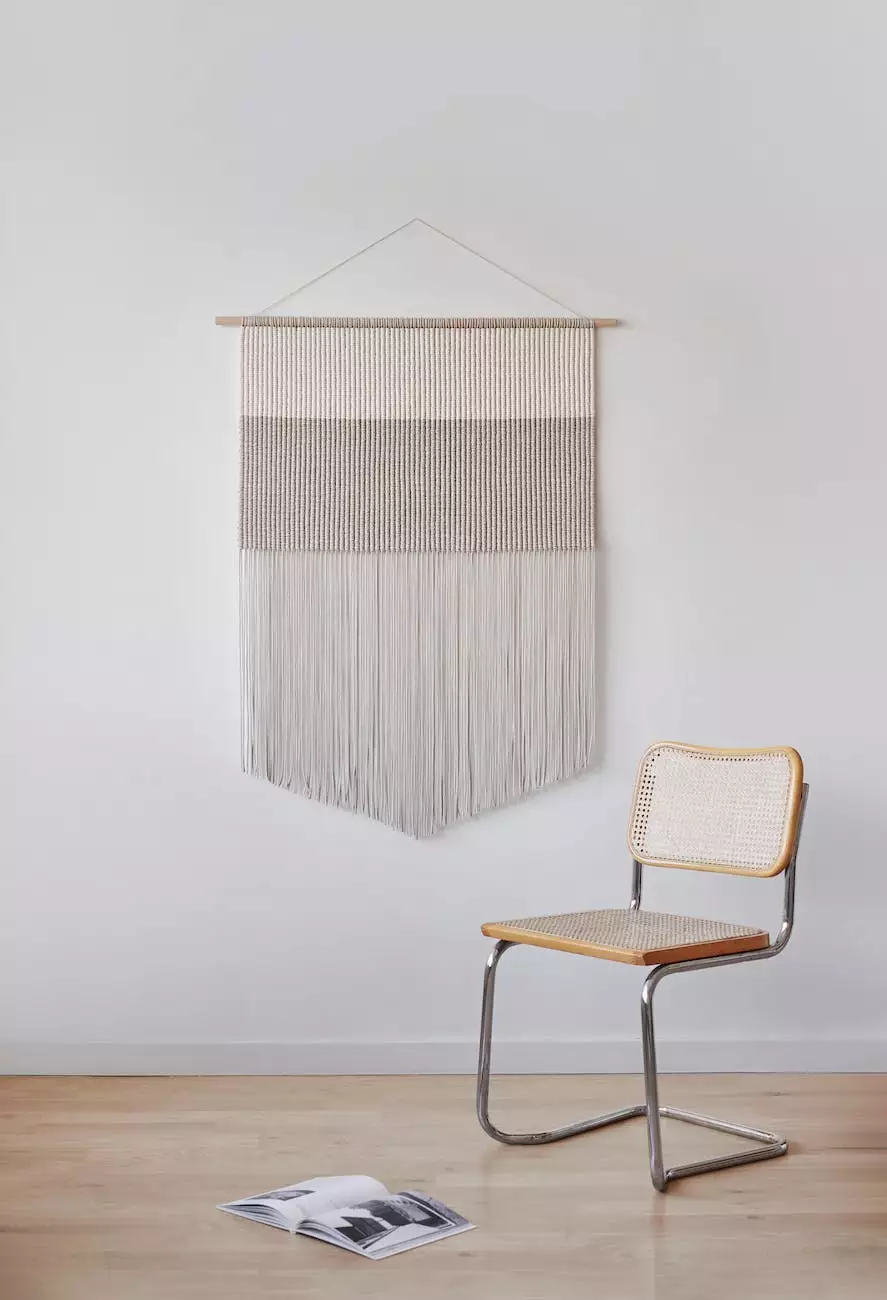
Welcome to Genevish Graphics! In this comprehensive guide, we will delve into one of the most common errors encountered by JavaScript developers - the 'Cannot Read Property 'style' of Null' error. Whether you are an experienced developer or just starting your journey, this resource is designed to provide you with an in-depth understanding of the issue and step-by-step solutions to fix it.
Understanding the Error
Before we dive into the fixes, it's important to understand what the 'Cannot Read Property 'style' of Null' error means. This error typically occurs when you try to access the 'style' property of a variable that is null or undefined. The 'style' property is commonly used to manipulate CSS styles, so if the variable is null, you won't be able to access its 'style' property, resulting in this error.
Common Causes of the Error
There are several common scenarios that can lead to the 'Cannot Read Property 'style' of Null' error:
- The element you are trying to access doesn't exist in the DOM.
- The element exists, but it hasn't finished loading when the JavaScript code runs.
- The JavaScript code is running before the HTML document is fully parsed.
- There is a typo or mistake in the code that assigns a null or undefined value to the variable.
Fixing the Error
Now, let's explore the various solutions to fix the 'Cannot Read Property 'style' of Null' error:
1. Check DOM Element Existence
To avoid accessing a null element, you should always check if the element exists in the DOM before manipulating its style:
const element = document.getElementById('myElement'); if (element) { // Access the style property here } else { console.error('Element not found.'); }2. Use window.onload Event
If the error occurs because the element hasn't finished loading, you can use the window.onload event to ensure that the JavaScript code runs only after the HTML document is fully loaded:
window.onload = function() { // Access the element's style property here };3. Place JavaScript Code After HTML
If the code is running before the HTML document is fully parsed, you can solve the issue by moving your JavaScript code below the HTML content:
My Website4. Debug the Code
If none of the above solutions work, it's possible that there is a mistake or typo in your code that causes the variable to be null or undefined. You can use browser tools like the JavaScript console to identify and fix the issue:
console.log(myVariable); // Check the variable's value // Make sure the variable is assigned a valid element const element = document.getElementById('myElement'); // Double-check that the element exists before accessing its style property if (element) { // Access the style property here } else { console.error('Element not found.'); }Conclusion
Congratulations! You have learned how to resolve the 'Cannot Read Property 'style' of Null' error in JavaScript. Remember to always perform checks and handle potential null values when accessing DOM elements to avoid encountering this common error. By applying the techniques outlined in this guide, you'll be able to write more robust and error-free JavaScript code.
We hope this resource has been helpful and informative. If you have any further questions or need additional assistance, feel free to browse our website and contact our team at Genevish Graphics. Good luck and happy coding!