How to Convert Map Values to an Array in JavaScript
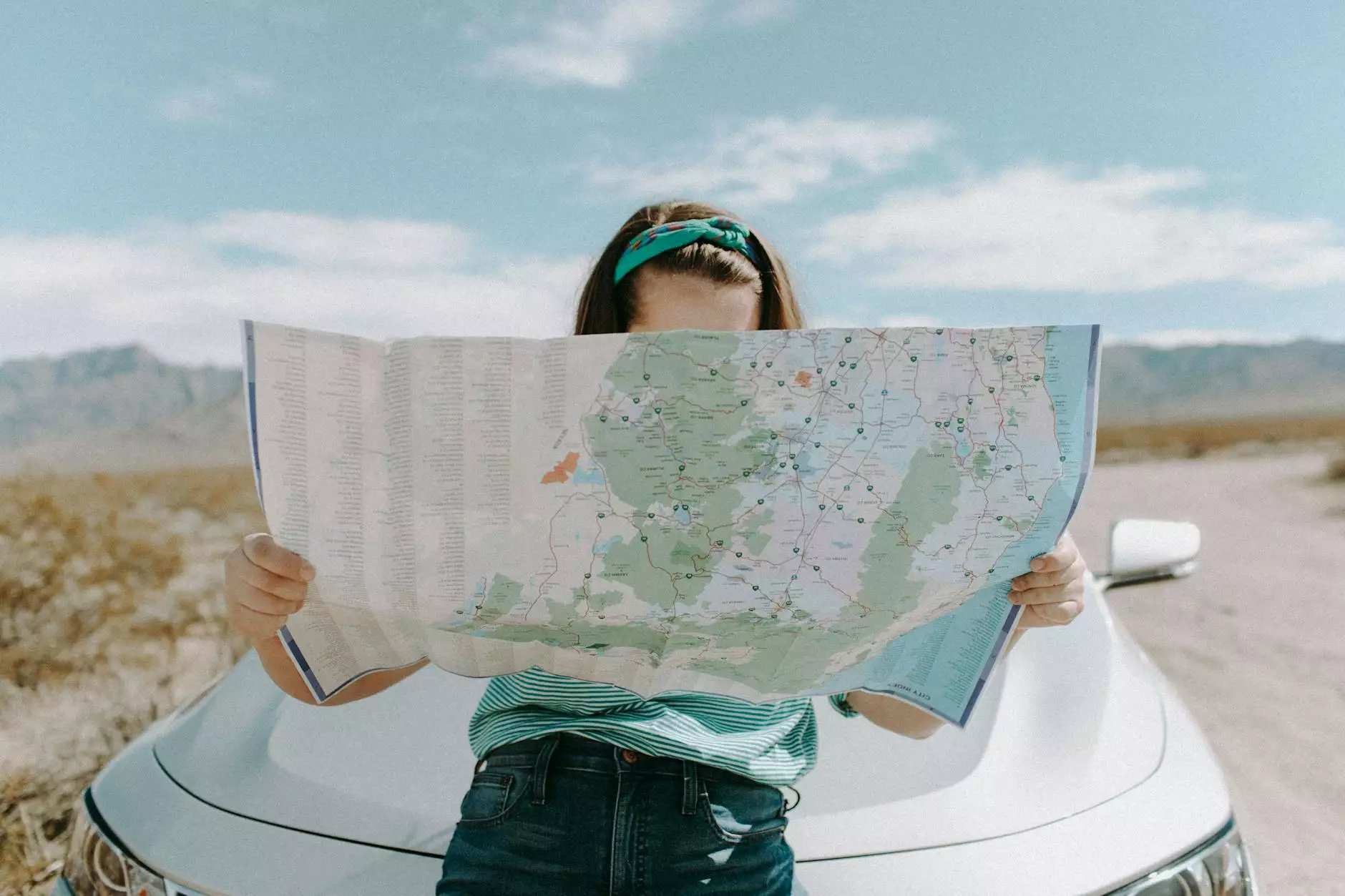
Introduction
Welcome to Genevish Graphics, your trusted source for arts and entertainment related to visual arts and design. In this latest article, we will explore how to convert map values to an array in JavaScript. JavaScript is a versatile programming language widely used for web development, and knowing how to manipulate map data is crucial for efficient code execution. By the end of this tutorial, you will have a clear understanding of the process and be able to apply it to your own projects.
Understanding Maps and Arrays
Before we dive into converting map values to an array, let's establish a solid understanding of what maps and arrays are in JavaScript.
A map in JavaScript is an object that stores key-value pairs. It allows you to associate unique keys with specific values. Maps are particularly useful when you need quick retrieval of values based on their corresponding keys. Think of it as a dictionary where you can easily look up definitions.
An array, on the other hand, is an ordered collection of items. Each item in an array is assigned an index, starting from 0. Arrays are commonly used for storing multiple values and provide various methods for accessing and manipulating the data efficiently.
Converting Map Values to an Array in JavaScript
Now, let's get down to business and learn how to convert map values to an array in JavaScript. Follow these step-by-step instructions closely to achieve the desired result.
Step 1: Create a Map
In order to convert map values to an array, we first need to create a map object. We can do this using the Map constructor in JavaScript. Here's an example:
const myMap = new Map();This will create an empty map named myMap. You can also initialize it with key-value pairs if needed.
Step 2: Adding Values to the Map
Now that we have our map, it's time to populate it with some values. You can add key-value pairs to the map using the set() method. Here's an example:
myMap.set('key1', 'value1'); myMap.set('key2', 'value2'); myMap.set('key3', 'value3');This adds three key-value pairs to the map, associating unique keys with specific values. Feel free to customize the keys and values based on your requirements.
Step 3: Converting Map Values to an Array
To convert the map values to an array, we can utilize the Array.from() method, which creates a new array instance from an iterable object. In this case, our map is the iterable object. Here's how you can convert the map values to an array:
const valuesArray = Array.from(myMap.values());Now, the valuesArray variable will contain an array with all the values from the map. You can access and manipulate this array just like any other array in JavaScript.
Step 4: Utilizing the Array of Map Values
Once you have the array of map values, you can perform various operations on it depending on your specific needs. Some common use cases include:
- Iterating over the values: You can use forEach() or for...of loop to iterate over the values and perform actions on each value individually.
- Performing calculations: If the values are numerical, you can use array methods such as reduce() or map() to perform calculations or transformations on the values.
- Filtering values: You can use array methods like filter() to selectively filter out values based on certain criteria.
- Anything else: The possibilities are endless with arrays. You can utilize various array methods and techniques to accomplish your specific goals.
Conclusion
Congratulations! You have successfully learned how to convert map values to an array in JavaScript. Understanding this process will greatly enhance your ability to manipulate map data effectively. You can now implement this knowledge in your own projects and optimize your code for better performance. Remember to experiment and explore other JavaScript techniques to broaden your skill set. Happy coding!