How to Check if an Object is Empty in JavaScript
Blog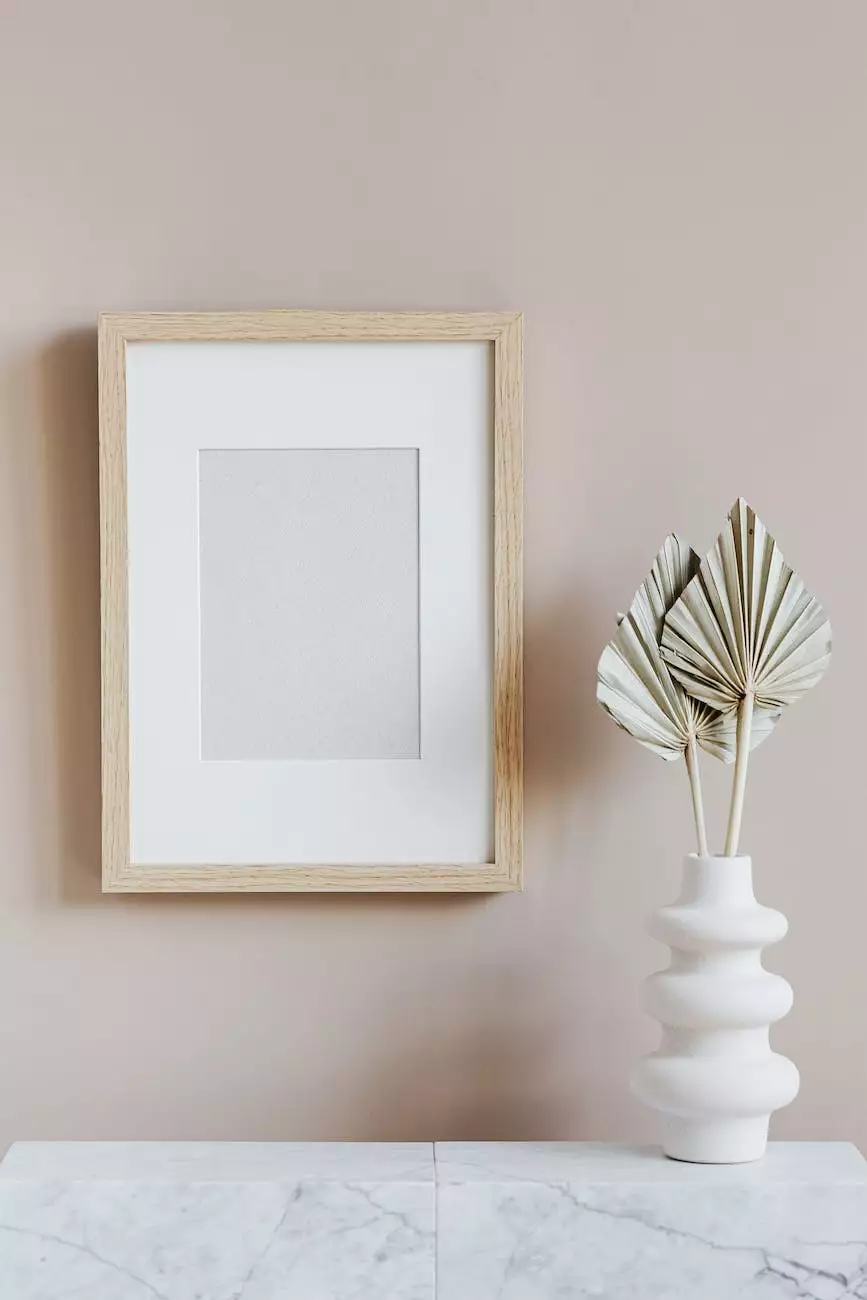
Welcome to Genevish Graphics, your go-to resource for all things arts and entertainment in the realm of visual arts and design. In this comprehensive guide, we will delve into the topic of checking if an object is empty in JavaScript, equipping you with the knowledge and techniques you need to confidently handle empty objects in your JavaScript code.
Understanding Empty Objects in JavaScript
Before we dive into the techniques for checking if an object is empty in JavaScript, let's first understand what constitutes an empty object. In JavaScript, an empty object is one that does not contain any properties, meaning it has no key-value pairs.
Method 1: Object.keys()
One way to determine if an object is empty is by making use of the Object.keys() method. This method returns an array of a given object's own enumerable property names, allowing us to check the length of the array to determine if the object is empty or not.
let obj = {}; let isEmpty = Object.keys(obj).length === 0;Method 2: Object.entries()
Another option is to use the Object.entries() method, which returns an array of a given object's own enumerable property [key, value] pairs. By checking the length of the resulting array, we can determine if the object is empty.
let obj = {}; let isEmpty = Object.entries(obj).length === 0;Method 3: Looping through Object Properties
If you prefer a more traditional approach, looping through the object's properties can also help determine if it is empty. By using a simple for...in loop, we can check if the object has any properties, and accordingly, conclude if it is empty or not.
let obj = {}; let isEmpty = true; for (let prop in obj) { if (obj.hasOwnProperty(prop)) { isEmpty = false; break; } }Method 4: JSON.stringify()
Alternatively, you can utilize the JSON.stringify() method to check if an object is empty. By converting the object to a JSON string and comparing it to an empty object representation, we can ascertain if the object is empty or not.
let obj = {}; let isEmpty = JSON.stringify(obj) === "{}";Conclusion
In this comprehensive guide, we explored various methods and techniques for checking if an object is empty in JavaScript. Whether you prefer using Object.keys(), Object.entries(), object property looping, or JSON.stringify(), it's important to choose the method that best suits your specific use case. Armed with this newfound knowledge, you can confidently handle and manipulate empty objects in your JavaScript code.
At Genevish Graphics, we strive to provide you with the most relevant and valuable information to enhance your arts and entertainment endeavors. Stay tuned for more insightful guides and tutorials!