How to Get the Class Name of an Object in JavaScript
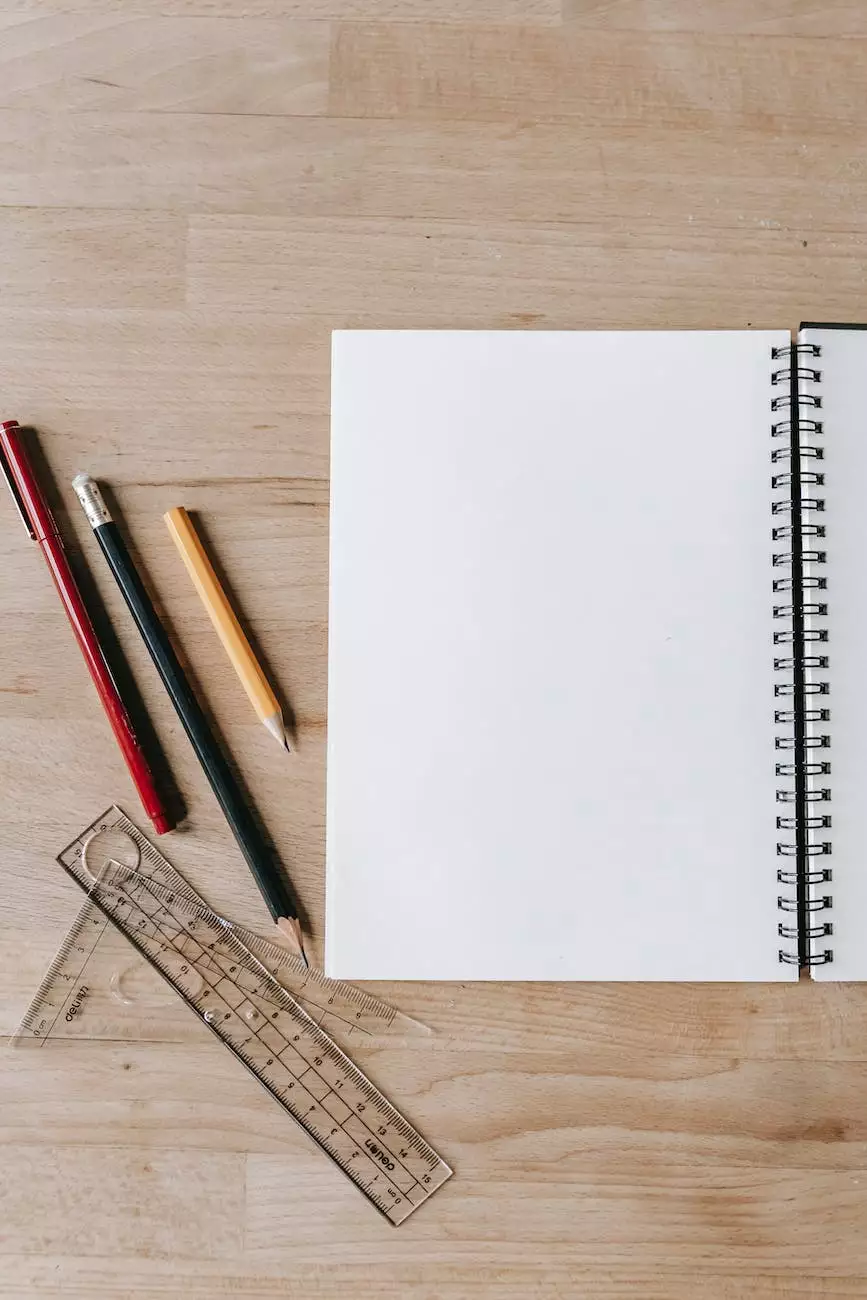
Introduction
Welcome to Genevish Graphics, your comprehensive resource for arts & entertainment in the field of visual arts and design. In this tutorial, we'll explore a common challenge faced by JavaScript developers - getting the class name of an object.
Understanding JavaScript Objects
Before we dive into getting the class name of an object, it's important to have a foundational understanding of JavaScript objects. In JavaScript, objects are a fundamental data structure that allows us to store and manipulate complex data. Objects are made up of properties and methods, which are essentially variables and functions respectively. They provide a way to organize and structure data in a meaningful way.
Why Do We Need to Know the Class Name of an Object?
Knowing the class name of an object can be incredibly useful in various scenarios. It allows us to perform specific actions or make decisions based on the type of object we are working with. For example, in a visual arts and design application, we might have different classes that represent different shapes. By knowing the class name, we can apply specific styling or functionality to each shape type.
Method 1: Using the constructor property
One way to get the class name of an object in JavaScript is by accessing the constructor property:
const obj = new SomeClass(); const className = obj.constructor.name; console.log(className); // Output: SomeClassMethod 2: Leveraging instanceof operator
Another approach is to use the instanceof operator, which allows us to check if an object is an instance of a specific class:
const obj = new SomeClass(); if (obj instanceof SomeClass) { console.log('Object belongs to SomeClass'); } else { console.log('Object does not belong to SomeClass'); }Method 3: Using Object.prototype.toString()
An alternative method involves using the toString() method from the Object.prototype. This method returns a string representation of the object, including the class name:
const obj = new SomeClass(); const className = Object.prototype.toString.call(obj).slice(8, -1); console.log(className); // Output: SomeClassChoosing the Right Method
Each of the methods mentioned above has its own advantages and considerations. The preferred method depends on the specific use case and the JavaScript environment you are working with. It's important to understand the differences and choose the most suitable approach for your project.
Conclusion
In conclusion, understanding how to get the class name of an object in JavaScript is essential for effective coding in the visual arts and design domain. Whether you choose to use the constructor property, the instanceof operator, or the toString() method, having this knowledge will enhance your ability to create dynamic and personalized experiences in your next arts & entertainment project.
About Genevish Graphics
Genevish Graphics is a leading online platform in the arts & entertainment industry. We specialize in providing valuable resources and tutorials to help individuals improve their skills in visual arts and design. Our team of experts is dedicated to delivering comprehensive content that caters to all skill levels.
Additional Resources
- Genevish Graphics - Explore our website for more informative articles and tutorials.
- Visual Arts and Design - Discover our extensive collection of visual arts and design resources.
- Tutorials - Expand your knowledge with our step-by-step tutorials.
- Blog - Stay updated with the latest trends and insights in the arts & entertainment industry.
- Contact - Have a question? Get in touch with our team.